This Posts Contains Complete Detailed Code to Generates a Shortcode that outputs the copyright notice for a website, including the copyright symbol, the current year, the name of the company, and the “All Rights Reserved” text.
If you want the text “Copyright © 2023 Company Name. All Rights Reserved.” to be updated to the current year Automatically in your WordPress footer, without any Plugin Installation, the we can Write a function that enables a Shortcode which can then simply be added in your Footer Widget. In this Post we will discuss a Generic Function in Functions.php File that Generates a Shortcode for Copyright © Current Year Company Name. All Rights Reserved. in the Footer Widget in your WordPress Theme.
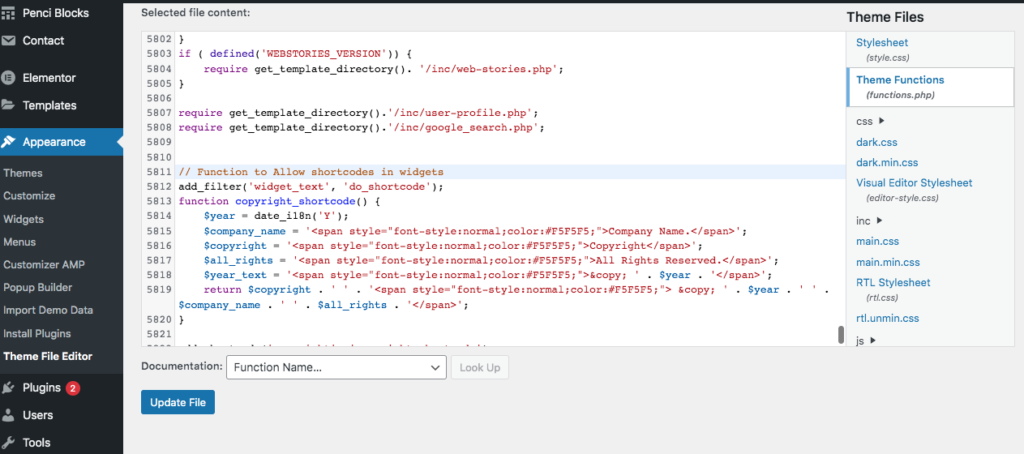
Function that Allows to Implement Automatic Year Update shortcode in Footer widget
add_filter(‘widget_text’, ‘do_shortcode’);
function copyright_shortcode()
{
$year = date_i18n(‘Y’); $company_name = ‘<span style=”font-style:normal;color:#F5F5F5;”>Company Name.</span>’; $copyright = ‘<span style=”font-style:normal;color:#F5F5F5;”>Copyright</span>’; $all_rights = ‘<span style=”font-style:normal;color:#F5F5F5;”>All Rights Reserved.</span>’; $year_text = ‘<span style=”font-style:normal;color:#F5F5F5;”>© ‘ . $year . ‘</span>’; return $copyright . ‘ ‘ . ‘<span style=”font-style:normal;color:#F5F5F5;”> © ‘ . $year . ‘ ‘ . $company_name . ‘ ‘ . $all_rights . ‘</span>’;
}
add_shortcode(‘copyright’, ‘copyright_shortcode’);
Code Explanation Step by Step:
function copyright_shortcode() {
This line declares a function named copyright_shortcode
.
$year = date_i18n('Y');
This line gets the current year using the WordPress function date_i18n()
and assigns it to a variable named $year
$company_name = ‘<span style=”font-style:normal;color:#F5F5F5;”>Company Name.</span>’;
This line defines a variable $company_name
and assigns it a value of a string, which is wrapped in a span
tag with some inline CSS styles. The styles set the font style to normal and the colour to a shade of greyish-white (#F5F5F5). The string itself is “Company Name.” with a period at the end.)
$copyright = ‘<span style=”font-style:normal;color:#F5F5F5;”>Copyright</span>’;
This line defines a variable $copyright
and assigns it a value of a string, which is wrapped in a span
tag with some inline CSS styles. The styles set the font style to normal and the colour to a shade of greyish-white (#F5F5F5). The string itself is “Copyright”.
$all_rights = ‘<span style=”font-style:normal;color:#F5F5F5;”>All Rights Reserved.</span>’;
This line defines a variable $all_rights
and assigns it a value of a string, which is wrapped in a span
tag with some inline CSS styles. The styles set the font style to normal and the color to a shade of greyish-white (#F5F5F5). The string itself is “All Rights Reserved.” with a period at the end.
$year_text = ‘<span style=”font-style:normal;color:#F5F5F5;”>© ‘ . $year . ‘</span>’;
This line defines a variable $year_text
and assigns it a value of a string, which is wrapped in a span
tag with some inline CSS styles. The styles set the font style to normal and the color to a shade of greyish-white (#F5F5F5). The string itself is an HTML entity for the copyright symbol (©
) followed by the current year, which is retrieved using the date_i18n()
function.
return $copyright . ‘ ‘ . ‘<span style=”font-style:normal;color:#F5F5F5;”> © ‘ . $year . ‘ ‘ . $company_name . ‘ ‘ . $all_rights . ‘</span>’;
return
statement is used to return the final output from the function.$copyright
is a string variable that contains the text “Copyright” with some styling.' '
is used to concatenate the strings with a space between them.<span style="font-style:normal;color:#F5F5F5;"> ©
is an HTML span element that adds some styling and contains the copyright symbol, which is represented by©
.$year
is a PHP variable that contains the current year, which is fetched using thedate_i18n()
function..
is used to concatenate the string variables with the HTML element.$company_name
is a string variable that contains the company name with some styling. The company name is enclosed within an anchor tag that links to a specific URL.$all_rights
is a string variable that contains the text “All Rights Reserved” with some styling.</span>
is used to close the opening<span>
tag.The final output is enclosed within another<span>
tag with some styling.
Now Finally Go to the Footer Widget of your WordPress Theme and simply add “copyright” in Square Brackets: [copyright]
Doing this Will Generate the Following as displayed in the Image.

To Write the Company Name in Different Colour and Link it Back to Your Website, Here is the Modified Code :
add_filter(‘widget_text’, ‘do_shortcode’);
function copyright_shortcode() {
$year = date_i18n(‘Y’);
//$company_name = ‘<span style=”font-style:normal;color:#F5F5F5;”>Company Name.</span>’;
$company_name = ‘<a href=”https://CompanyWebsiteName./” style=”color: #FFA500; text-decoration: none;”>Company Name.</a>’;
$copyright = ‘<span style=”font-style:normal;color:#F5F5F5;”>Copyright</span>’;
$all_rights = ‘<span style=”font-style:normal;color:#F5F5F5;”>All Rights Reserved.</span>’;
$year_text = ‘<span style=”font-style:normal;color:#F5F5F5;”>© ‘ . $year . ‘</span>’;
return $copyright . ‘ ‘ . ‘<span style=”font-style:normal;color:#F5F5F5;”> © ‘ . $year . ‘ ‘ . $company_name . ‘ ‘ . $all_rights . ‘</span>’;
}
add_shortcode(‘copyright’, ‘copyright_shortcode’);
Step-by-step Explanation of the code:
function copyright_shortcode() {
: This line declares a function namedcopyright_shortcode
.$year = date_i18n('Y');
: This line gets the current year using the WordPress functiondate_i18n()
and assigns it to a variable named$year
.$company_name = '<a href="https://companywebsitename.com/" style="color: #FFA500; text-decoration: none;">Company Name</a>';
: This line sets the value of$company_name
to a hyperlink that links to YOUR COMPANY WEBSITE and has a colour of orange (#FFA500) and no text decoration.$copyright = '<span style="font-style:normal;color:#F5F5F5;">; Copyright</span>';
: This line sets the value of$copyright
to a span element with a normal font style and a color of greyish white (#F5F5F5), with the copyright symbol (©) followed by the word “COPYRIGHT”.$all_rights = '<span style="font-style:normal;color:#F5F5F5;">All Rights Reserved</span>';
: This line sets the value of$all_rights
to a span element with a normal font style and a color of greyish white (#F5F5F5), with the text “All Rights Reserved”.$year_text = '<span style="font-style:normal;color:#F5F5F5;">© ' . $year . '</span>';
: This line sets the value of$year_text
to a span element with a normal font style and a color of greyish white (#F5F5F5), with the copyright symbol (©) followed by the current year.return $copyright . ' ' . $year_text . ' ' . $company_name . '. ' . $all_rights;
: This line concatenates the values of$copyright
,$year_text
,$company_name
, and$all_rights
, separated by spaces and with a period at the end, and returns the resulting string.add_shortcode('copyright', 'copyright_shortcode');
: This line registers thecopyright_shortcode()
function as a shortcode with the name “copyright”.
Overall, the code generates a Shortcode that outputs the copyright notice for a website, including the copyright symbol, the current year, the name of the company, and the “All Rights Reserved” text, with the company name being a hyperlink to a specified website and with all text styled in a greyish white color. The Output looks like below :
